This microcontroller module is great as it is cheap, but also incorporates an OLED display. I admit, that the screen is extremely small 0.42″, but it can still display some basic information, and you will not buy a screen and the microcontroller for the price of ($6 or 5.6 EUR).
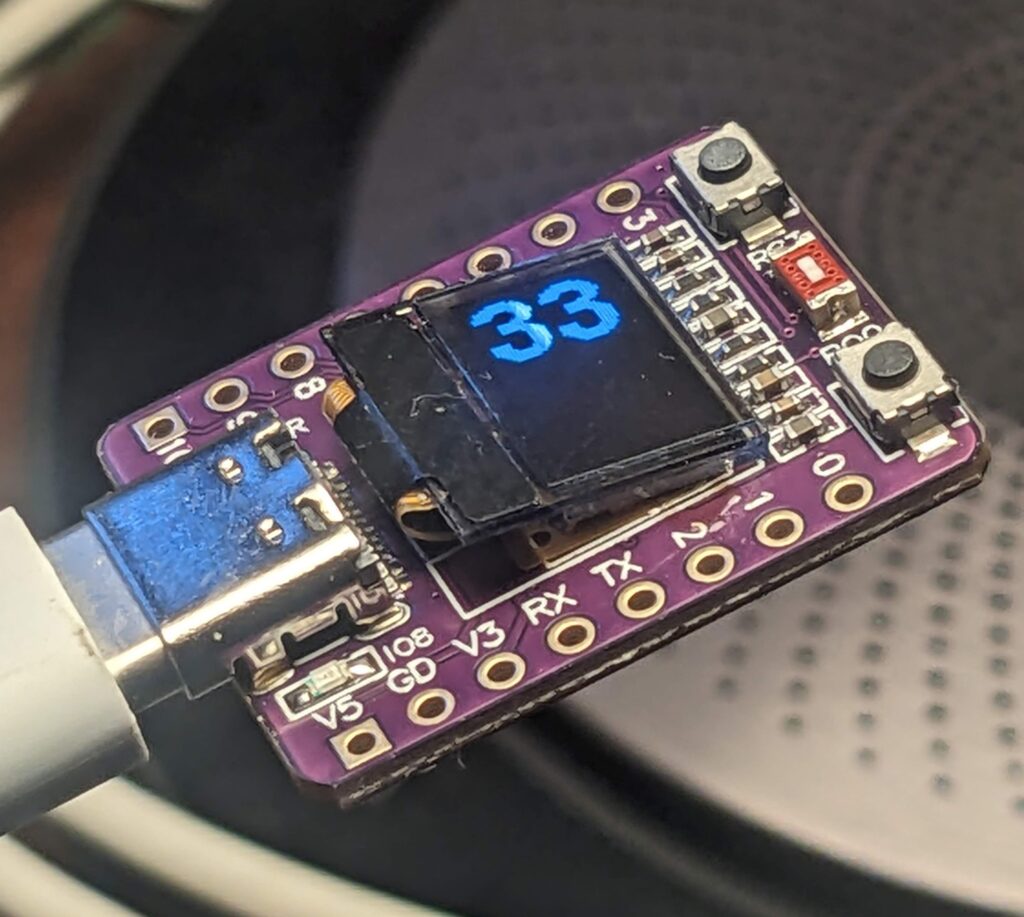
There are at least two variants out there with this name, on mine the buttons are located oppose from the USB connector.
Buy here
- https://s.click.aliexpress.com/e/_oD4R12v Should be around 5€ or $5.4
(Affiliate link)
Setting up in PlatformIO
We should use the espressif32
platform with the board of esp32-c3-devkitm-1
, however e.g. the LED_BUILTIN is not the same with my board, so this is not the same board layout.
[env:esp32-c3-oled]
platform = espressif32
board = esp32-c3-devkitm-1
framework = arduino
lib_deps =
olikraus/U8g2
Initial code
Here is a sample code for the module. It of course already tests the screen with the U8g2 library, as it is the star of the show. To list all the peripherals tested:
- On-board 72×40 OLED screen using hardware i2c,
- Built in LED on pin 8,
- Button called “BOOT” on pin 9,
- ESP32-c3 internal temperature sensor.
(We use some legacy code for the temperature sensing, so it will not work in the future.)
#include <Arduino.h>
#include <U8g2lib.h>
// Legacy library for the temperature sensor
#include "driver/temp_sensor.h"
#include <Wire.h>
#define SDA_PIN 5
#define SCL_PIN 6
#define PIN_LED 8
#define PIN_BUTTON 9
U8G2_SSD1306_72X40_ER_F_HW_I2C u8g2(U8G2_R0, U8X8_PIN_NONE);
void drawText(const char *text)
{
u8g2.drawStr(0, 0, text);
}
// Initialize the temperature sensor
void initTempSensor(){
temp_sensor_config_t temp_sensor = TSENS_CONFIG_DEFAULT();
temp_sensor.dac_offset = TSENS_DAC_L2;
temp_sensor_set_config(temp_sensor);
temp_sensor_start();
}
void setup() {
pinMode(PIN_LED, OUTPUT);
pinMode(PIN_BUTTON, INPUT);
Wire.begin(SDA_PIN, SCL_PIN);
u8g2.begin();
u8g2.setDisplayRotation(U8G2_R3); // Rotate the display 180 degrees
u8g2.setFont(u8g2_font_fub25_tf); // Use high pixel wide font
u8g2.setDrawColor(1);
u8g2.setFontPosTop();
initTempSensor();
}
char buffer[5];
void loop() {
// Read temperature and convert it to const char*
float temp_celsius = 0;
temp_sensor_read_celsius(&temp_celsius);
snprintf(buffer, sizeof(buffer), "%.0f", temp_celsius);
const char* buffer_c = buffer;
u8g2.clearBuffer();
drawText(buffer_c);
u8g2.sendBuffer();
digitalWrite(PIN_LED, digitalRead(PIN_BUTTON));
delay(100);
}
How to continue
We should also check
- WiFi and Bluetooth capability